

Active GUI element
Static GUI element
Code
WPS object
File/Path
Command line
Entry-field content
[Key combination]
Spell check in EPM with easily changeable dictionary files
The extended editor (EPM.EXE) is an universal tool which is not only suitable for processing simple text files but, e. g., as a front-end for LaTeX.
The integrated dictionary function can be applied to spell checking the LaTeX source text. Apart from the possibility of checking the *.DVI file with ISPELL, it is more convenient to use the integrated function in EPM because necessary corrections can be made immediately in the editor. The dictionary files distributed with IBMWORKS (Bonuspak), e.g., US.DCT, have proven usable.
The possibility arose of easily switching between different dictionary configurations in EPM, especially between old and new German orthography, or even other languages.
E is the macro language for IBM's EPM editor.
- An example
- An EDM/2 article: Customizing the Enhanced Editor
It is unrelated to The Programming Language E.
Since the programming of additional menu calls—including the appropriate routines with the macro language E—for EPM is not a problem, it also seemed obvious to add a menu item for switching the currently used dictionary file together with the appropriate user generated dictionary files.
The goals for the version presented here are:
- no new entries in the file EPM.INI must be created
- no recompilation of the file EPM.EX is necessary
- the overhead for the administration of several dictionary files should be as small as possible
Creating a Dictionary Menu
With a correctly installed spell-checking support (for the English version: Options > Preferences > Settings > Paths) the dictionary adjustments are recorded by EPM in the file EPM.INI.
With the following source code, placed in SwitchDict.E
, the switching function can be integrated into the
menu.
The Constants
First we need a definition for text constants which appear in the status line when the new functions are called:
const DICT_BAR_MSG = 'Modifying dictionary files' ActDicMSG = 'Switch to dictionary file: ' definit universal defaultmenu, APP_HINI, DICTIONARY_FILENAME, ADDENDA_FILENAME, Dict_ID, Add_ID, SwDict
The procedures in definit
are executed at the start of EPM
(more exactly when each new window is opened) and contain the default values.
The access to variables and constants used in EPM is done via the
keyword universal
.
The Variables
The definition of global variables which are accessible by multiple procedures is also possible. One needs:
Variable | Description |
---|---|
Internal variables | |
defaultmenu | handle to the standard menu |
APP_HINI | handle to the file EPM.INI |
DICTIONARY_FILENAME | internal variable of EPM, contains the path and file name of the dictionary |
ADDENDA_FILENAME | internal variable EPM, contains the path and file name of the supplement dictionary created by the user |
New variables | |
Dict_ID | defined variable, field index for dictionary files |
Add_ID | defined variable, field index for supplemental dictionary files |
SwDict | defined variable, switch for current files and index for array variables |
For the access to the different files with a single procedure, it is best to use an array structure. Arrays in the language E require a bit of work.
The transfer to the arrays can only take place via variables which are assigned the paths to the dictionary files. The paths must be assigned according to the current environment. The inclusion of these default settings into the menu structure of EPM is possible. It does, however, require additional administration effort, e.g., additional entries in the EPM.INI.
It is convenient to use a HOME directory for the storage of the dictionary files.
Hom = get_env('home') Dic1 = HOM||'\DEUTSCH.DIC' Dic2 = HOM||'\DEUTSCH2.DIC' Dic3 = HOM||'US.DCT' Add1 = HOM||'\DEUTSCH.ADL' Add2 = HOM||'\DEUTSCH2.ADL' Add3 = HOM||'\US.ADL' Dic1 = 'C:\BONUSPAK\IBMWORKS\DEUTSCH.DIC' Dic2 = 'C:\BONUSPAK\IBMWORKS\DEUTSCH2.DIC' Dic3 = 'C:\LEXAM\US.DCT' Add1 = 'C:\BONUSPAK\IBMWORKS\DEUTSCH.ADL' Add2 = 'C:\BONUSPAK\IBMWORKS\DEUTSCH2.ADL' Add3 = 'C:\LEXAM\US.ADL' do_array 1, Dict_ID, 'Dict' do_array 2, Dict_ID, '1', Dic1 do_array 2, Dict_ID, '2', Dic2 do_array 2, Dict_ID, '3', Dic3 do_array 1, Add_ID, 'Add' do_array 2, Add_ID, '1', Add1 do_array 2, Add_ID, '2', Add2 do_array 2, Add_ID, '3', Add3
The path of the current dictionary file is derived from the keyword DICTIONARY
in
EPM.INI and the variable SwDict is set to that value. The value of SwDict is needed for the
indexing the array variables and for indication in the menu.
ActvDict = queryprofile(APP_HINI,'EPM','DICTIONARY') if ActvDict = Dic1 then SwDict = 1 elseif ActvDict = Dic2 then SwDict = 2 elseif ActvDict = Dic3 then SwDict = 3 endif
Defining the Menu Items
For the remake of the whole menu the Help menu is deleted temporarily:
deletemenu defaultmenu, 6, 0, 0
The inclusion of the new submenu is done as follows:
buildsubmenu defaultmenu,2000,'~Dictionary',\1DICT_BAR_MSG,0x31,0 buildmenuitem defaultmenu,2000,2001,'Deutsch-Alt','SetDic 1'\1ActDicMSG Dic1,0,0 buildmenuitem defaultmenu,2000,2002,'Deutsch-Neu','SetDic 2'\1ActDicMSG Dic2,0,0 buildmenuitem defaultmenu,2000,2003,'US-English','SetDic 3'\1ActDicMSG Dic3,0,0
The identifications 2000. . .2003 are necessary for EPM to distinguish between the structures and needn't
be numbered as shown. (That is taken into account with further menu expansions.) The command which is executed after
the selection of the designated submenu item is called SetDict
here and is defined later. After
this command a comment follows which contains the constant ActDicMSG. The comment is displayed in
the message window of EPM during the selection of the appropriate submenu-item.
The style of the submenu is defined by the second to last value in the "defaultmenu" list, in this case, 0x31; it is the logical OR of these constants:
MIS_TEXT | MIS_SUBDMENU | MIS_MULTMENU = 0x31
. The inclusion of the appropriate header file from the [OS/2] programming toolkit (pmwin.h)
allows the use of symbolic constants such as MIS_TEXT
.
The last value in the list, 0 (zero), represents the attribute which is set during the menu-initialization.
With the inclusion of the appropriate header-files from the programming toolkit (PMWIN.H), it is also possible to use the symbolic constants.
After adding the Help menu:
call readd_help_menu()
the whole menu is displayed:
showmenu defaultmenu
If more dictionaries (three in this example) are required, the array variables and submenus have to be expanded accordingly.
Menu Actions
The next part is defining of the routine to execute when the submenu item is selected:
defc SetDic universal APP_HINI, DICTIONARY_FILENAME, ADDENDA_FILENAME, SwDict, Dict_ID, Add_ID
Access to the paths stored in the array variables is by assigning the array argument to the variable SwDict:
SwDict = Arg(1) do_array 7, Dict_ID, SwDict, Dict do_array 7, Add_ID, SwDict, ADD .
Not only will both paths be assigned to the variables used by EPM's current session, they are also stored in the EPM.INI to make the setting persistent.
DICTIONARY_FILENAME = Dict ADDENDA_FILENAME = Add SetProfile(APP_HINI,'EPM','DICTIONARY',Dict) SetProfile(APP_HINI,'EPM','ADDENDA',ADD)
With the selection of the new submenu item EPM executes the following routine which ensures here that the
selected language is marked with a CHECK label. The attribute MIA_CHECKED
= 0x2000
is set with the internal routine SetMenuAttribute
(defined in STDCTRL.E) in such way that in each case a selection is marked. A prerequisite for this is that the
menu style already set with the definition repeated here:
MIS_TEXT|MIS_SUBDMENU|MIS_MULTMENU = 0x31. defc menuinit_2000 universal SwDict SetMenuAttribute(2000, 0x31, 1) SetMenuAttribute(2001, 0x2000, SwDict<>1) SetMenuAttribute(2002, 0x2000, SwDict<>2) SetMenuAttribute(2003, 0x2000, SwDict<>3)
Putting it All Together
After the adjustments according to the paths this source code can be compiled with the ETPM.EXE into a binary form:
ETPM SwitchDict.E
Integration of the new function after compiling is done by adding a line to the file PROFILE.ERX:
' link SwitchDict.EX '
If REXX profile support is enabled in EPM (Options > Adjustment > Adjustments > Different REXX profile), the new point of submenu is available (see Fig. 1).
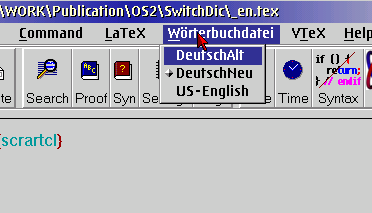
Figure 1. EPM with changeable dictionary files.
The specification of false paths in the file SwitchDict.E causes only an error message of EPM. After a correction a new compilation of SwitchDict.E is naturally necessary.
Final Notes
The available code was developed for my own applications and can surely be still optimized or developed further. Also the direct inclusion into the file MYSTUFF.E is conceivable.
With a new compilation of the entire EPM environment by ETPM EPM.E a new file EPM.EX is produced, which contains this supplement and the inclusion by PROFILE.ERX is not necessary.
For introduction to EPM programming the articles in the former OS/2 Inside Magazine [1] ... [5] should be mentioned apart from the examples in the package EPM603b.ZIP.